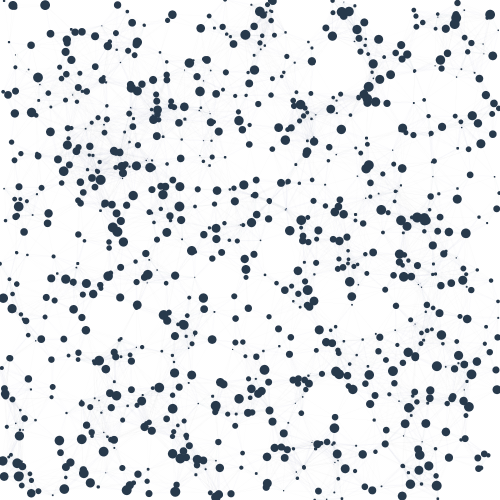
Processingソースコード(.pde)
static final int NUM_PRATICLES = 500;
Particle [] particles = new Particle[NUM_PRATICLES];
void setup() {
size(500, 500);
frameRate(30);
for(int i = 0; i < NUM_PRATICLES; i++){
particles[i] = new Particle();
}
}
void draw() {
background(255);
pushMatrix();
for (int i = 0; i < particles.length; i++) {
particles[i].moveMe();
particles[i].joinParticles(particles);
particles[i].drawMe();
}
translate(width/2, height/2);
popMatrix();
}
class Particle {
float x, y;
float r;
float xSpeed, ySpeed;
float xAcceleration, yAcceleration;
float alpha;
Particle() {
x = random(0, width);
y = random(0, height);
r = random(1, 10);
xSpeed = random(-2, 2);
ySpeed = random(-2, 2);
alpha = random(25, 250);
xAcceleration = 0.0;
yAcceleration = 0.0;
}
void drawMe() {
noStroke();
fill(39,57,75);
circle(x, y, r);
}
void moveMe() {
if (x < 0 || width < x) {
xSpeed *= -1;
}
if (y < 0 || height < y) {
ySpeed *= -0.975;
}
x += xSpeed;
y += ySpeed;
xAcceleration = (width/2 - x) / width;
yAcceleration = (height/2 - y) / height;
xSpeed += xAcceleration;
ySpeed += yAcceleration;
}
void joinParticles(Particle particles[]) {
for (int i = 0; i < particles.length; i++) {
float distance = dist(x, y, particles[i].x, particles[i].y);
if (distance < 50) {
stroke(39, 57, 75, (50 - distance) / 10);
line(x, y, particles[i].x, particles[i].y);
}
}
}
}